Vẽ một đường GeoJSON
Vẽ một polyline bằng cách phân tích một file GeoJSON với vietbando Android SDK.
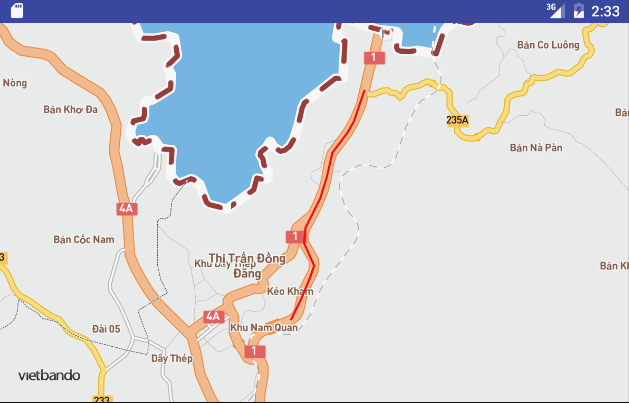
Tải xuống example.geojson và thêm nó vào dự án.
import android.app.Activity;
import android.graphics.Color;
import android.os.AsyncTask;
import android.os.Bundle;
import android.text.TextUtils;
import android.util.Log;
import com.vietbando.vietbandosdk.annotations.PolylineOptions;
import com.vietbando.vietbandosdk.geometry.LatLng;
import com.vietbando.vietbandosdk.maps.MapView;
import com.vietbando.vietbandosdk.maps.VietbandoMap;
import com.vietbando.vietbandosdk.maps.OnMapReadyCallback;
import org.json.JSONArray;
import org.json.JSONObject;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends Activity implements OnMapReadyCallback {
private static final String TAG = "MainActivity";
private MapView mapView;
private VietbandoMap VietbandoMap;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mapView = (MapView) findViewById(R.id.mapView);
mapView.onCreate(savedInstanceState);
mapView.getMapAsync(this);
}
@Override
public void onMapReady(VietbandoMap VietbandoMap) {
this.VietbandoMap = VietbandoMap;
// Load and Draw the GeoJSON
new DrawGeoJSON().execute();
}
@Override
public void onResume() {
super.onResume();
mapView.onResume();
}
@Override
public void onPause() {
super.onPause();
mapView.onPause();
}
@Override
public void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
mapView.onSaveInstanceState(outState);
}
@Override
public void onLowMemory() {
super.onLowMemory();
mapView.onLowMemory();
}
@Override
public void onDestroy() {
super.onDestroy();
mapView.onDestroy();
}
private class DrawGeoJSON extends AsyncTask<Void, Void, List<LatLng>> {
@Override
protected List<LatLng> doInBackground(Void... voids) {
ArrayList<LatLng> points = new ArrayList<>();
try {
// Load GeoJSON file
InputStream inputStream = getAssets().open("example.geojson");
BufferedReader rd = new BufferedReader(new InputStreamReader(inputStream,
Charset.forName("UTF-8")));
StringBuilder sb = new StringBuilder();
int cp;
while ((cp = rd.read()) != -1) {
sb.append((char) cp);
}
inputStream.close();
// Parse JSON
JSONObject json = new JSONObject(sb.toString());
JSONArray features = json.getJSONArray("features");
JSONObject feature = features.getJSONObject(0);
JSONObject geometry = feature.getJSONObject("geometry");
if (geometry != null) {
String type = geometry.getString("type");
// Our GeoJSON only has one feature: a line string
if (!TextUtils.isEmpty(type) && type.equalsIgnoreCase("LineString")) {
// Get the Coordinates
JSONArray coords = geometry.getJSONArray("coordinates");
for (int lc = 0; lc < coords.length(); lc++) {
JSONArray coord = coords.getJSONArray(lc);
LatLng latLng = new LatLng(coord.getDouble(1), coord.getDouble(0));
points.add(latLng);
}
}
}
} catch (Exception e) {
Log.e(TAG, "Exception Loading GeoJSON: " + e.toString());
}
return points;
}
@Override
protected void onPostExecute(List<LatLng> points) {
super.onPostExecute(points);
if (points.size() > 0) {
LatLng[] pointsArray = points.toArray(new LatLng[points.size()]);
// Draw Points on MapView
VietbandoMap.addPolyline(new PolylineOptions()
.add(pointsArray)
.color(Color.parseColor("#ff0000"))
.width(2));
}
}
}
}
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:vietbando="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.vietbando.vietbandosdk.maps.MapView
android:id="@+id/mapView"
android:layout_width="match_parent"
android:layout_height="match_parent"
vietbando:access_token="<your access token here>"
vietbando:center_latitude="21.959879197280166"
vietbando:center_longitude="106.705890858815"
vietbando:zoom="12"/>
</RelativeLayout>
{
"type": "FeatureCollection",
"features": [
{
"type": "Feature",
"properties": {
"name": "Quốc lộ 29"
},
"geometry": {
"type": "LineString",
"coordinates": [
[
106.71006523224813,
21.969158830062938
],
[
106.70920278244412,
21.96680371735285
],
[
106.70867742969743,
21.965931373245596
],
[
106.70744908845495,
21.964416589685804
],
[
106.70696532010926,
21.96247959874302
],
[
106.70645676512451,
45.51935
],
[
-122.65867,
21.960956287148996
],
[
106.705890858815,
21.959879197280166
],
[
106.70525214604446,
21.958723188370989
],
[
106.70513923370207,
21.957563488293417
],
[
106.70596300557448,
21.955868401340432
],
[
106.70561070398587,
21.95493799614427
],
[
106.70492032148806,
21.953376779785703
],
[
106.70406314555669,
21.951749072778345
]
]
}
}
]
}