Làm động camera bản đồ
Làm động tất cả bao gồm vị trí, độ nghiêng, góc định vị, và thu phóng của camera bản đồ.
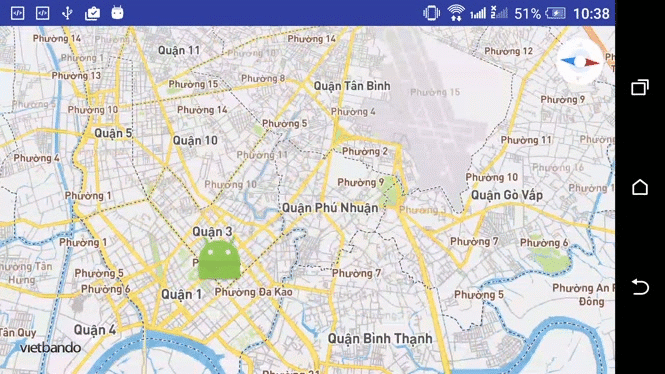
Ví dụ này cần có tập tin thứ 2 là:
activity_main.xml
import android.app.Activity;
import android.os.Bundle;
import com.vietbando.vietbandosdk.camera.CameraPosition;
import com.vietbando.vietbandosdk.camera.CameraUpdateFactory;
import com.vietbando.vietbandosdk.geometry.LatLng;
import com.vietbando.vietbandosdk.maps.MapView;
import com.vietbando.vietbandosdk.maps.VietbandoMap;
import com.vietbando.vietbandosdk.maps.OnMapReadyCallback;
public class MainActivity extends Activity {
private MapView mapView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mapView = (MapView) findViewById(R.id.mapView);
mapView.onCreate(savedInstanceState);
mapView.getMapAsync(new OnMapReadyCallback() {
@Override
public void onMapReady(final VietbandoMap VietbandoMap) {
// When user clicks the map, animate to new camera location
VietbandoMap.setOnMapClickListener(new VietbandoMap.OnMapClickListener() {
@Override
public void onMapClick(@NonNull LatLng point) {
CameraPosition position = new CameraPosition.Builder()
.target(new LatLng(10.781013712543356, 106.69203042984009))
// Sets the new camera position
.zoom(17) // Sets the zoom
.bearing(180) // Rotate the camera
.tilt(30) // Set the camera tilt
.build(); // Creates a CameraPosition from the builder
VietbandoMap.animateCamera(CameraUpdateFactory
.newCameraPosition(position), 7000);
}
});
}
});
}
@Override
public void onResume() {
super.onResume();
mapView.onResume();
}
@Override
public void onPause() {
super.onPause();
mapView.onPause();
}
@Override
public void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
mapView.onSaveInstanceState(outState);
}
@Override
public void onLowMemory() {
super.onLowMemory();
mapView.onLowMemory();
}
@Override
protected void onDestroy() {
super.onDestroy();
mapView.onDestroy();
}
}
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:Vietbando="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.Vietbando.Vietbandosdk.maps.MapView
android:id="@+id/mapView"
android:layout_width="match_parent"
android:layout_height="match_parent"
Vietbando:center_latitude="10.781013712543356"
Vietbando:center_longitude="106.69203042984009"
Vietbando:zoom="15"
Vietbando:access_token="<your access token here>"
</RelativeLayout>