Vẽ một marker tùy chỉnh
Thêm một marker bản đồ với hình ảnh tùy chỉnh trong Vietbando iOS SDK.
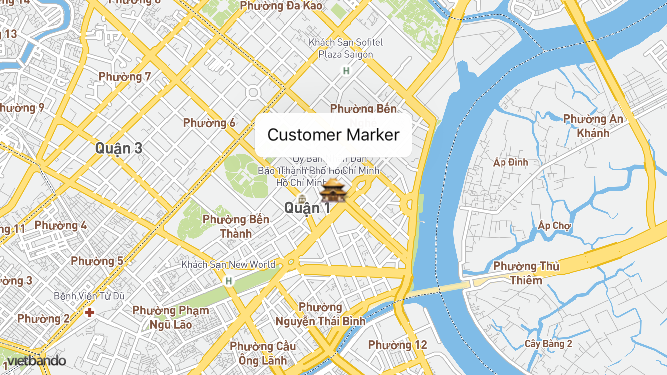
Tải về ảnh biểu tượng agoda và thêm nó vào dự án.
#import "ViewController.h"
@import Vietbando;
@interface ViewController () <VBDMapViewDelegate>
@end
@implementation ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
VBDMapView *mapView = [[VBDMapView alloc] initWithFrame:self.view.bounds];
mapView.autoresizingMask = UIViewAutoresizingFlexibleWidth | UIViewAutoresizingFlexibleHeight;
mapView.tintColor = [UIColor darkGrayColor];
// Set the map view‘s delegate property
mapView.delegate = self;
mapView setCenterCoordinate:CLLocationCoordinate2DMake(10.7763973, 106.701278)
zoomLevel:13
animated:NO];
// Initialize and add the marker annotation
VBDPointAnnotation *agoda = [[VBDPointAnnotation alloc] init];
agoda.coordinate = CLLocationCoordinate2DMake(10.7763973, 106.701278);
agoda.title = @"Customer Marker";
[mapView addAnnotation:agoda];
}
- (VBDAnnotationImage *)mapView:(VBDMapView *)mapView imageForAnnotation:(id <VBDAnnotation>)annotation
{
// Try to reuse the existing ‘agoda’ annotation image, if it exists
VBDAnnotationImage *annotationImage = [mapView dequeueReusableAnnotationImageWithIdentifier:@"agoda"];
// If the ‘agoda’ annotation image hasn‘t been set yet, initialize it here
if ( ! annotationImage)
{
// Customer marker from the Noun Project
UIImage *image = [UIImage imageNamed:@"agoda"];
// The anchor point of an annotation is currently always the center. To
// shift the anchor point to the bottom of the annotation, the image
// asset includes transparent bottom padding equal to the original image
// height.
//
// To make this padding non-interactive, we create another image object
// with a custom alignment rect that excludes the padding.
image = [image imageWithAlignmentRectInsets:UIEdgeInsetsMake(0, 0, image.size.height/2, 0)];
// Initialize the ‘agoda’ annotation image with the UIImage we just loaded
annotationImage = [VBDAnnotationImage annotationImageWithImage:image reuseIdentifier:@"agoda"];
}
return annotationImage;
}
- (BOOL)mapView:(VBDMapView *)mapView annotationCanShowCallout:(id <VBDAnnotation>)annotation {
// Always allow callouts to popup when annotations are tapped
return YES;
}
@end