Tìm đường đi
Đây là một trong các dịch vụ do Vietbando cung cấp, giúp nguời dùng tìm đường đi một cách nhanh chóng và chính xác trong phạm vi 63 tỉnh thành trong cả nước.
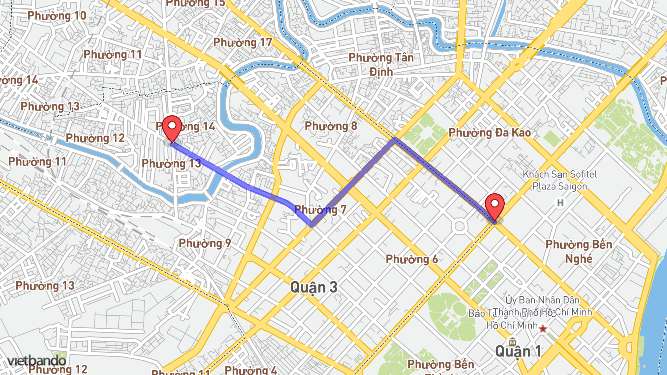
Tải về thư viện Vietbando Services.
#import "ViewController.h"
@import Vietbando;
@import VietbandoServices;
@interface ViewController ()
@property (nonatomic) IBOutlet VBDMapView *mapView;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
}
- (void)viewWillAppear:(BOOL)animated{
if ([VBDAccountManager accessToken].length == 0)
{
UIAlertController*alertController = [UIAlertController alertControllerWithTitle:@"Register Key" message:@"Enter your Vietbando Register Key:" preferredStyle:UIAlertControllerStyleAlert];
[alertController addTextFieldWithConfigurationHandler:^(UITextField * _Nonnull textField)
{
textField.keyboardType = UIKeyboardTypeURL;
textField.autocorrectionType = UITextAutocorrectionTypeNo;
textField.autocapitalizationType = UITextAutocapitalizationTypeNone;
}];
[alertController addAction:[UIAlertAction actionWithTitle:@"Cancel" style:UIAlertActionStyleCancel handler:nil]];
UIAlertAction *OKAction = [UIAlertAction actionWithTitle:@"OK" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action)
{
UITextField *textField = alertController.textFields.firstObject;
NSString *accessToken = textField.text;
[VBDAccountManager setAccessToken:accessToken];
[self.mapView reloadStyle:self];
[self Routing];
}];
[alertController addAction:OKAction];
[self presentViewController:alertController animated:YES completion:nil];
}
else{
[self Routing];
}
}
//#pragma mark Direction
- (void) Routing{
CLLocationCoordinate2D points[2] = {
CLLocationCoordinate2DMake(10.7829318,106.698102),
CLLocationCoordinate2DMake(10.7876535,106.678276)
};
VBDPointAnnotation *pS = [[VBDPointAnnotation alloc] init];
pS.coordinate = points[0];
pS.title = @"Điểm bắt đầu";
[self.mapView addAnnotation:pS];
VBDPointAnnotation *pE = [[VBDPointAnnotation alloc] init];
pE.coordinate = points[1];
pE.title = @"Điểm kết thúc";
[self.mapView addAnnotation:pE];
NSString *sRegisterKey = [VBDAccountManager accessToken];
[VBDServices Direction:points num:2 registerKey:sRegisterKey
success:^(id obj) {
NSDictionary *json = (NSDictionary *)obj;
if( json && [json isKindOfClass:[NSDictionary class]]){
NSMutableArray *fullPath = [json objectForKey:@"FullPath"];
NSMutableArray *path = [fullPath objectAtIndex:0];
NSArray *points = [path objectAtIndex:0];
int numberOfCoordinates = (int)[points count]/2;
// unpacking an array of NSValues into memory
CLLocationCoordinate2D *coordinates = malloc(numberOfCoordinates * sizeof(CLLocationCoordinate2D));
for(int i = 0; i < numberOfCoordinates; i++) {
int index = i*2;
double lon = [[points objectAtIndex:index] doubleValue];
double lat = [[points objectAtIndex:index+1] doubleValue];
coordinates[i] = CLLocationCoordinate2DMake( lat, lon);
}
[self drawShape:coordinates numberOfCoordinates:numberOfCoordinates];
free(coordinates);
}
else{
[self showError:@"Không tìm thấy!"];
}
}
fail:^(id obj) {
[self showError:(NSString *)obj];
}
];
}
- (void) showError:(NSString *)errorText{
UIAlertController*alertController= [UIAlertController alertControllerWithTitle:@"Error" message:errorText preferredStyle:UIAlertControllerStyleAlert];
UIAlertAction* ok = [UIAlertAction actionWithTitle:@"OK" style:UIAlertActionStyleDefault handler:nil];
[alertControlleraddAction:ok];
[self presentViewController:alertController animated:YES completion:nil];
}
#pragma mark draw shape
- (void)drawShape:(CLLocationCoordinate2D *)coordinates numberOfCoordinates:(NSUInteger)numberOfCoordinates
{
// Create our shape with the formatted coordinates array
VBDPolyline *shape = [VBDPolyline polylineWithCoordinates:coordinates count:numberOfCoordinates];
// Add the shape to the map
[self.mapView addAnnotation:shape];
[self.mapView setVisibleCoordinates:coordinates count:numberOfCoordinates edgePadding:UIEdgeInsetsMake(25, 10, 45, 10) animated:YES];
}
- (CGFloat)mapView:(VBDMapView *)mapView lineWidthForPolylineAnnotation:(VBDPolyline *)annotation
{
// Set the line width for polyline annotations
return 6.0f;
}
- (CGFloat)mapView:(VBDMapView *)mapView alphaForShapeAnnotation:(VBDShape *)annotation
{
// Set the alpha for shape annotations to 0.5 (half opacity)
return 0.5f;
}
- (UIColor *)mapView:(VBDMapView *)mapView strokeColorForShapeAnnotation:(VBDShape *)annotation
{
// Set the stroke color for shape annotations
return [UIColor blueColor];
}
- (UIColor *)mapView:(VBDMapView *)mapView fillColorForPolygonAnnotation:(VBDPolygon *)annotation
{
// Mapbox cyan fill color
return [UIColor colorWithRed:59.0f/255.0f green:178.0f/255.0f blue:208.0f/255.0f alpha:1.0f];
}
@end