Tìm xung quanh
Từ một vị trí đã chọn trên bản đồ, người dùng có thể tìm kiếm các dịch vụ xung quanh vị trí đó như: trường học, bệnh viện, nhà hàng, khách sạn, quán ăn, dịch vụ massage,….
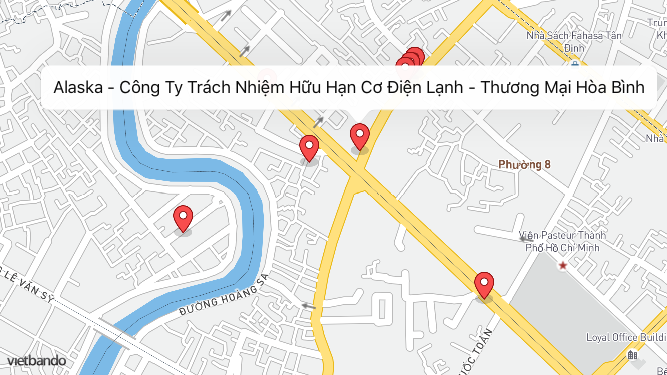
Tải về thư viện Vietbando Services.
#import "ViewController.h"
#define KEY_SEARCH @"Hotel"
@import Vietbando;
@import VietbandoServices;
@interface ViewController ()
@property (nonatomic) IBOutlet VBDMapView *mapView;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)viewWillAppear:(BOOL)animated{
if ([VBDAccountManager accessToken].length == 0)
{
UIAlertController*alertController = [UIAlertControlleralertControllerWithTitle:@"Register Key" message:@"Enter your Vietbando Register Key:" preferredStyle:UIAlertControllerStyleAlert];
[alertController addTextFieldWithConfigurationHandler:^(UITextField * _Nonnull textField)
{
textField.keyboardType = UIKeyboardTypeURL;
textField.autocorrectionType = UITextAutocorrectionTypeNo;
textField.autocapitalizationType = UITextAutocapitalizationTypeNone;
}];
[alertController addAction:[UIAlertAction actionWithTitle:@"Cancel" style:UIAlertActionStyleCancel handler:nil]];
UIAlertAction *OKAction = [UIAlertAction actionWithTitle:@"OK" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action)
{
UITextField *textField = alertController.textFields.firstObject;
NSString *accessToken = textField.text;
[VBDAccountManager setAccessToken:accessToken];
[self.mapView reloadStyle:self];
[self SearchNearBy:KEY_SEARCH radius:[NSNumber numberWithInt:1000]];
}];
[alertController addAction:OKAction];
[self presentViewController:alertController animated:YES completion:nil];
}
else{
[self SearchNearBy:KEY_SEARCH radius:[NSNumber numberWithInt:1000]];
}
}
- (void) SearchNearBy:(NSString *)key radius:(NSNumber *)radius
{
NSString *sRegisterKey = [VBDAccountManager accessToken];
CLLocationCoordinate2D centerCoordinate = CLLocationCoordinate2DMake(10.7871580999995, 106.685230000001);
[VBDServices SearchNearBy:key page:[NSNumber numberWithInt:1] pagesize:[NSNumber numberWithInt:10] centerCoordinate:centerCoordinate radius:[NSNumber numberWithInt:1000] registerKey:sRegisterKey
success:^(id obj){
NSArray *list = (NSArray *)obj;
if( list && [list isKindOfClass:[NSArray class]] && [list count]>0 ){
NSMutableArray *annos = [[NSMutableArray alloc] init];
for( NSDictionary *dic in list )
{
VBDPointAnnotation *anno = [[VBDPointAnnotation alloc] init];
double lat = [[dic objectForKey:@"Latitude"] doubleValue];
double lon = [[dic objectForKey:@"Longitude"] doubleValue];
anno.coordinate = CLLocationCoordinate2DMake(lat,lon);
anno.title = [dic objectForKey:@"Name"];
[annos addObject:anno];
}
[self.mapView addAnnotations:annos];
[self.mapView showAnnotations:annos animated:YES];
}
else{
[self showError:@"Không tìm thấy!"];
}
}
fail:^(id obj) {
[self showError:(NSString *)obj];
}
];
}
- (void) showError:(NSString *)errorText{
UIAlertController*alertController = [UIAlertControlleralertControllerWithTitle:@"Error" message:errorText preferredStyle:UIAlertControllerStyleAlert];
UIAlertAction* ok = [UIAlertAction actionWithTitle:@"OK" style:UIAlertActionStyleDefault handler:nil];
[alertController addAction:ok];
[self presentViewController:alertController animated:YES completion:nil];
}
#pragma mark VBDMapView
// Use the default marker; see our custom marker example for more information
- (VBDAnnotationImage *)mapView:(VBDMapView *)mapView imageForAnnotation:(id )annotation {
return nil;
}
// Allow markers callouts to show when tapped
- (BOOL)mapView:(VBDMapView *)mapView annotationCanShowCallout:(id )annotation {
return YES;
}
@end